Check out JetpackCompose.app's Newsletter - Dispatch for Android and Jetpack Compose updates
SUBSCRIBE NOW![User avatar]()
I always learn something just by skimming it that makes me want to bookmark the issue now and dig deeper later

SUBSCRIBE NOW![User avatar]()
Keep up the good work with the newsletter 💪 I really enjoy it

SUBSCRIBE NOW![User avatar]()
Dispatch is a must read for Android devs today and my go-to for keeping up with all things Jetpack Compose

SUBSCRIBE NOW![User avatar]()
Dispatch has been my go-to resource as it's packed with useful information while being fun at the same time

SUBSCRIBE NOW![User avatar]()
The content is light, fun, and still useful. I especially appreciate the small tips that are in each issue

SUBSCRIBE NOW![User avatar]()
I truly love this newsletter ❤️🔥 Spot on content and I know there's a lot of effort that goes behind it

SUBSCRIBE NOW![User avatar]()
Thanks for taking the time and energy to do it so well

JetpackCompose.app's Newsletter![User avatar]()
I always learn something just by skimming it that makes me want to bookmark the issue now and dig deeper later

JetpackCompose.app's Newsletter![User avatar]()
Keep up the good work with the newsletter 💪 I really enjoy it

JetpackCompose.app's Newsletter![User avatar]()
Dispatch is a must read for Android devs today and my go-to for keeping up with all things Jetpack Compose

JetpackCompose.app's Newsletter![User avatar]()
Dispatch has been my go-to resource as it's packed with useful information while being fun at the same time

JetpackCompose.app's Newsletter![User avatar]()
The content is light, fun, and still useful. I especially appreciate the small tips that are in each issue

JetpackCompose.app's Newsletter![User avatar]()
I truly love this newsletter ❤️🔥 Spot on content and I know there's a lot of effort that goes behind it

JetpackCompose.app's Newsletter![User avatar]()
Thanks for taking the time and energy to do it so well

Floating Action Button with Material Animations
Author: Johan Reitan
Floating Action Button with animations that are implemented as per the Material Design spec.
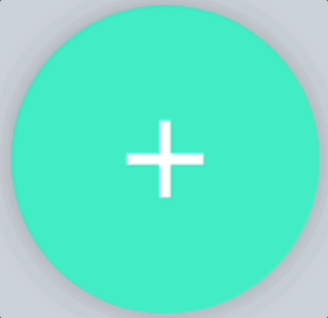
Have a project you'd like to submit? Fill this form, will ya!
If you like this snippet, you might also like:
Maker OS is an all-in-one productivity system for developers
I built Maker OS to track, manage & organize my life. Now you can do it too!
© 2025 All Rights Reserved | Made by Vinay Gaba